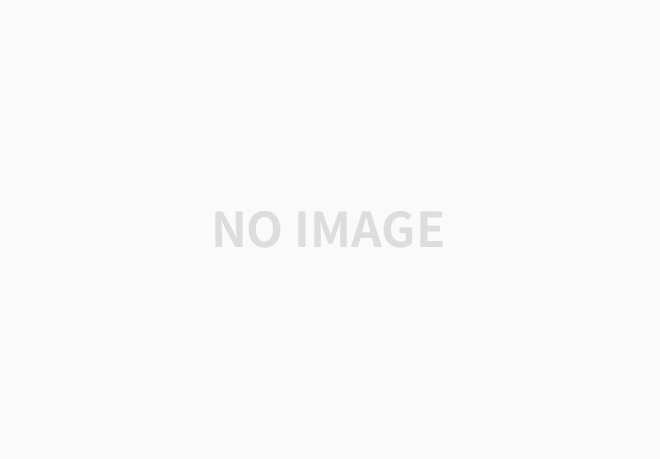
1. 石头剪刀布游戏
- 概念:玩家与计算机进行石头剪刀布对决。玩家选择一项,计算机随机选择,判断胜负。
- 技术:使用`Math.random()`生成随机数来代表计算机的选择。
- 代码示例:
```javascript
function getComputerChoice() {
const choices = ['rock', 'paper', 'scissors'];
const randomIndex = Math.floor(Math.random() * choices.length);
return choices[randomIndex];
}
function playGame(playerChoice) {
const computerChoice = getComputerChoice();
if (playerChoice === computerChoice) {
return "It's a tie!";
} else if (
(playerChoice === 'rock' && computerChoice === 'scissors') ||
(playerChoice === 'scissors' && computerChoice === 'paper') ||
(playerChoice === 'paper' && computerChoice === 'rock')
) {
return "You win!";
} else {
return "You lose!";
}
}
console.log(playGame('rock')); // Example usage
```
2. 猜数字游戏
- 概念:计算机生成一个随机的数字,玩家通过输入来猜测这个数字。
- 技术:使用`prompt`获取用户输入,并与生成的随机数进行比较。
- 代码示例:
```javascript
const targetNumber = Math.floor(Math.random() * 100) + 1;
let guess = null;
while (guess !== targetNumber) {
guess = parseInt(prompt('Guess a number between 1 and 100:'), 10);
if (guess < targetNumber) {
alert('Too low!');
} else if (guess > targetNumber) {
alert('Too high!');
} else {
alert('Congratulations! You guessed the right number.');
}
}
```
3. 简单的打地鼠游戏
- 概念:显示一个简单的界面,地鼠随机出现,玩家点击它们得分。
- 技术:使用`setInterval`和`addEventListener`来随机显示地鼠,并注册点击事件。
- 代码示例:
```javascript
const holes = document.querySelectorAll('.hole');
const scoreBoard = document.querySelector('.score');
let lastHole;
let score = 0;
function randomTime(min, max) {
return Math.round(Math.random() * (max - min) + min);
}
function randomHole(holes) {
const index = Math.floor(Math.random() * holes.length);
const hole = holes[index];
if (hole === lastHole) {
return randomHole(holes);
}
lastHole = hole;
return hole;
}
function peep() {
const time = randomTime(200, 1000);
const hole = randomHole(holes);
hole.classList.add('up');
setTimeout(() => {
hole.classList.remove('up');
if (!timeUp) peep();
}, time);
}
holes.forEach(hole => hole.addEventListener('click', () => {
if (!hole.classList.contains('up')) return;
score++;
scoreBoard.textContent = score;
hole.classList.remove('up');
}));
```
4. 简易记忆卡片游戏
- 概念:玩家翻看不同的卡片,匹配相同的一对,完成游戏。
- 技术:利用`array`管理卡片状态,用`setTimeout`给玩家查看的时间。
- 代码示例:
```javascript
const cardsArray = ['A', 'B', 'C', 'D', 'A', 'B', 'C', 'D'];
let firstCard, secondCard;
let hasFlippedCard = false;
let lockBoard = false;
function flipCard(card) {
if (lockBoard) return;
if (card === firstCard) return;
card.classList.add('flip');
if (!hasFlippedCard) {
hasFlippedCard = true;
firstCard = card;
return;
}
secondCard = card;
checkForMatch();
}
function checkForMatch() {
let isMatch = firstCard.dataset.framework === secondCard.dataset.framework;
isMatch ? disableCards() : unflipCards();
}
function disableCards() {
firstCard.removeEventListener('click', flipCard);
secondCard.removeEventListener('click', flipCard);
resetBoard();
}
function unflipCards() {
lockBoard = true;
setTimeout(() => {
firstCard.classList.remove('flip');
secondCard.classList.remove('flip');
resetBoard();
}, 1500);
}
function resetBoard() {
[hasFlippedCard, lockBoard] = [false, false];
[firstCard, secondCard] = [null, null];
}
// Assume card elements are correctly set with event listeners
```
这些示例应能为你提供开发JavaScript小游戏的一些思路和示范,帮助你开始自己的项目!