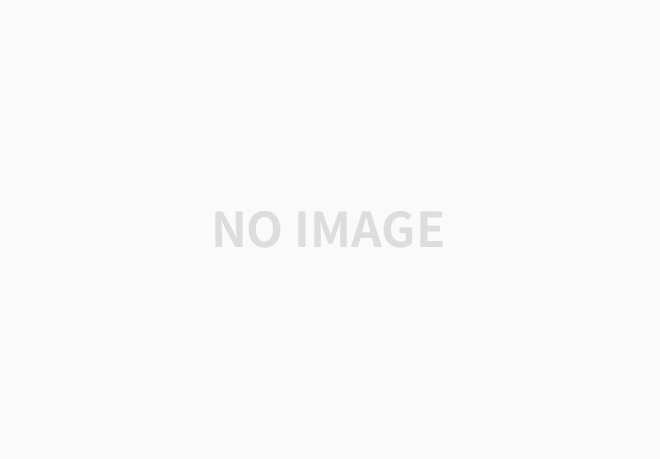
在Spring Boot应用程序中,了解API的数量对于监控和维护至关重要。在这篇文章中,我将详细讲解如何通过不同方法来识别和统计Spring Boot应用程序中的API数量。
首先,我们可以通过使用Spring的`RequestMappingHandlerMapping`类来获取所有API的映射信息。此方法无需额外的依赖,并且可以直接集成在Spring Boot应用程序中。下面是一个简单的示例来展示如何实现这一点:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
import org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping;
@Component
public class ApiCountRunner implements CommandLineRunner {
@Autowired
private RequestMappingHandlerMapping requestMappingHandlerMapping;
@Override
public void run(String... args) throws Exception {
int apiCount = requestMappingHandlerMapping.getHandlerMethods().size();
System.out.println("Total API count: " + apiCount);
}
}
```
在这个示例中,我们使用`CommandLineRunner`接口来在应用程序启动时输出API的总数。通过注入`RequestMappingHandlerMapping`,我们可以获取所有映射的handler方法,这些方法代表了API的数目。
其次,我们也可以通过Swagger来查看API数量。Swagger不仅提供了一个可视化界面来查看API文档,也可以用于统计API数量。假设你已经配置好Swagger,在浏览器中打开Swagger UI,界面通常会直接显示所有API的数量。
为此,我们需要确保Swagger已在项目中正确配置。以下是如何使用Swagger注解的一些基本示例:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
}
```
通过这种配置,你能在Swagger UI中轻松看到API的总数以及详细信息。
最后,还可以利用Spring Boot Actuator来帮助监控API统计数据。Spring Boot Actuator提供了丰富的端点数据,其中的`mappings`端点可以帮助我们检查和统计API。但是,需要确保启用了该功能。
在`application.properties`中启用端点:
```properties
management.endpoints.web.exposure.include=*
```
然后,你可以通过访问`/actuator/mappings`端点来查看详细的API列表和统计信息。
总结,以上几种方法都可以帮助你有效地统计和查看Spring Boot应用程序中的API数量。根据项目的需求和现状,你可以选择合适的方法来实施。