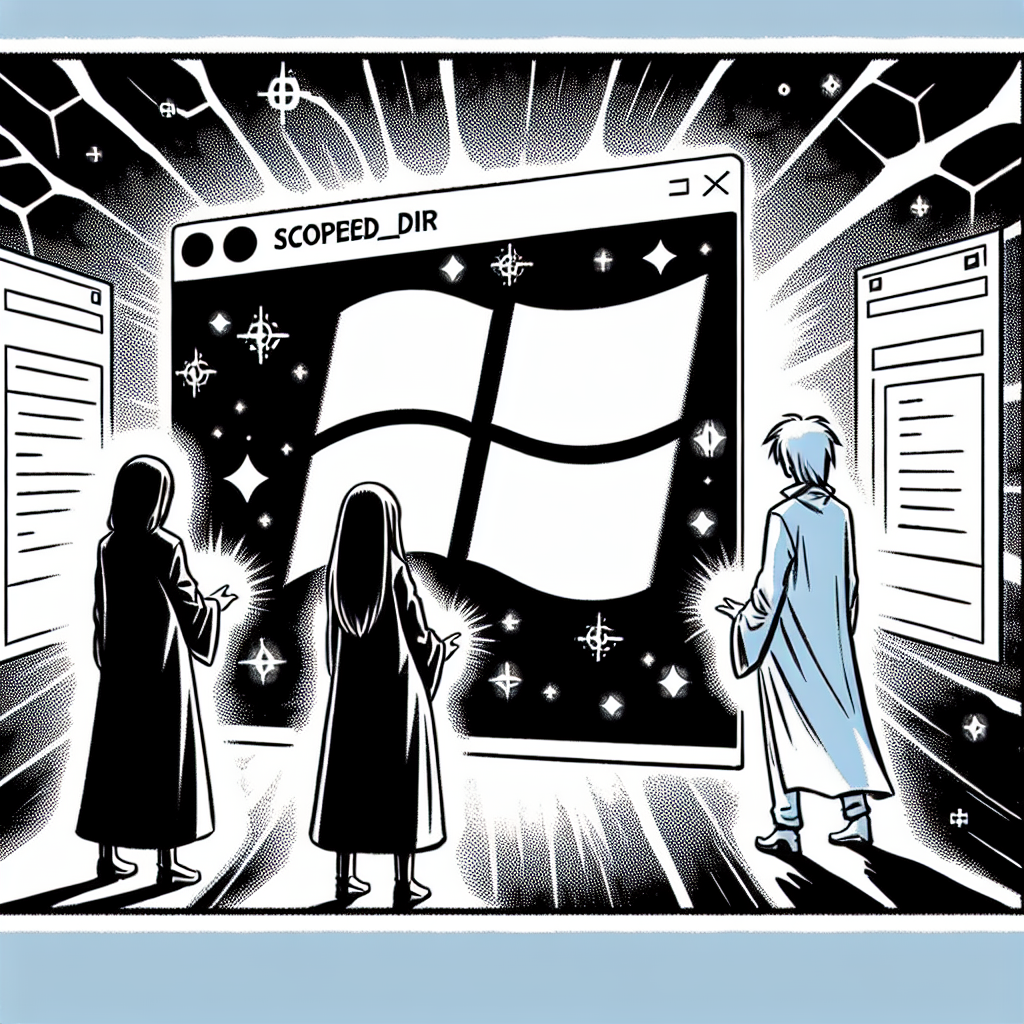
### Scoped Directory의 작동 방식
"scoped_dir"은 일반적으로 특정 범위 내에서만 유효한 임시 디렉토리를 제공하여, 프로세스가 끝나거나 파일이 더 이상 필요 없을 때 자동으로 삭제됩니다. 이는 주로 Windows API를 통해 사용되며, 개발자는 `CreateDirectory`와 `RemoveDirectory` 함수 등을 사용하여 수동으로 관리할 필요가 없습니다.
예제를 통해 사용법을 알아보겠습니다. 간단한 C++ 코드에서 임시 디렉토리를 생성하고 파일을 작성, 사용 후 자동 삭제하는 과정을 보여줍니다.
```cpp
#include <iostream>
#include <windows.h>
int main() {
// Get temporary file path
char tempPath[MAX_PATH];
GetTempPathA(MAX_PATH, tempPath);
// Create a scoped directory
char scopedDir[MAX_PATH];
GetTempFileNameA(tempPath, "scoped", 0, scopedDir);
CreateDirectoryA(scopedDir, NULL);
std::cout << "Scoped directory created at: " << scopedDir << std::endl;
// Do some file operations inside the scoped directory
// e.g., creating a temporary file
char tempFile[MAX_PATH];
sprintf_s(tempFile, "%s\\example.txt", scopedDir);
HANDLE hFile = CreateFileA(tempFile, GENERIC_WRITE, 0, NULL, CREATE_NEW, FILE_ATTRIBUTE_TEMPORARY, NULL);
if (hFile != INVALID_HANDLE_VALUE) {
const char* data = "Temporary data\n";
DWORD written;
WriteFile(hFile, data, strlen(data), &written, NULL);
CloseHandle(hFile);
std::cout << "Temporary file created and data written." << std::endl;
}
// Scoped directory will be removed at the end of program scope
RemoveDirectoryA(scopedDir);
std::cout << "Scoped directory removed." << std::endl;
return 0;
}
```
이 예제에서는 임시 디렉토리를 생성하고 그 안에 파일을 만든 다음, 데이터를 기록합니다. 프로그램의 실행이 끝나면 임시 디렉토리를 정리합니다.
### Scoped Directory의 장점
1. **자동 정리:** Scoped Directory는 더 이상 필요하지 않을 때 자동으로 정리되어 개발자의 관리 부담을 줄여줍니다.
2. **안전성:** 임시 데이터가 남아서 잠재적 보안 문제가 되는 것을 방지합니다.
3. **효율성:** 사용이 끝난 후 자동으로 정리되므로 디스크 공간 관리에 효율적입니다.
Windows의 scoped_dir 기능은 다양한 개발 환경에서 임시 데이터를 효율적으로 관리하는 데 유용하며, 위와 같은 방법으로 쉽게 구현할 수 있습니다. 적절한 활용으로 시스템 자원을 효과적으로 관리하고, 프로그램의 안전성과 성능을 높일 수 있습니다.