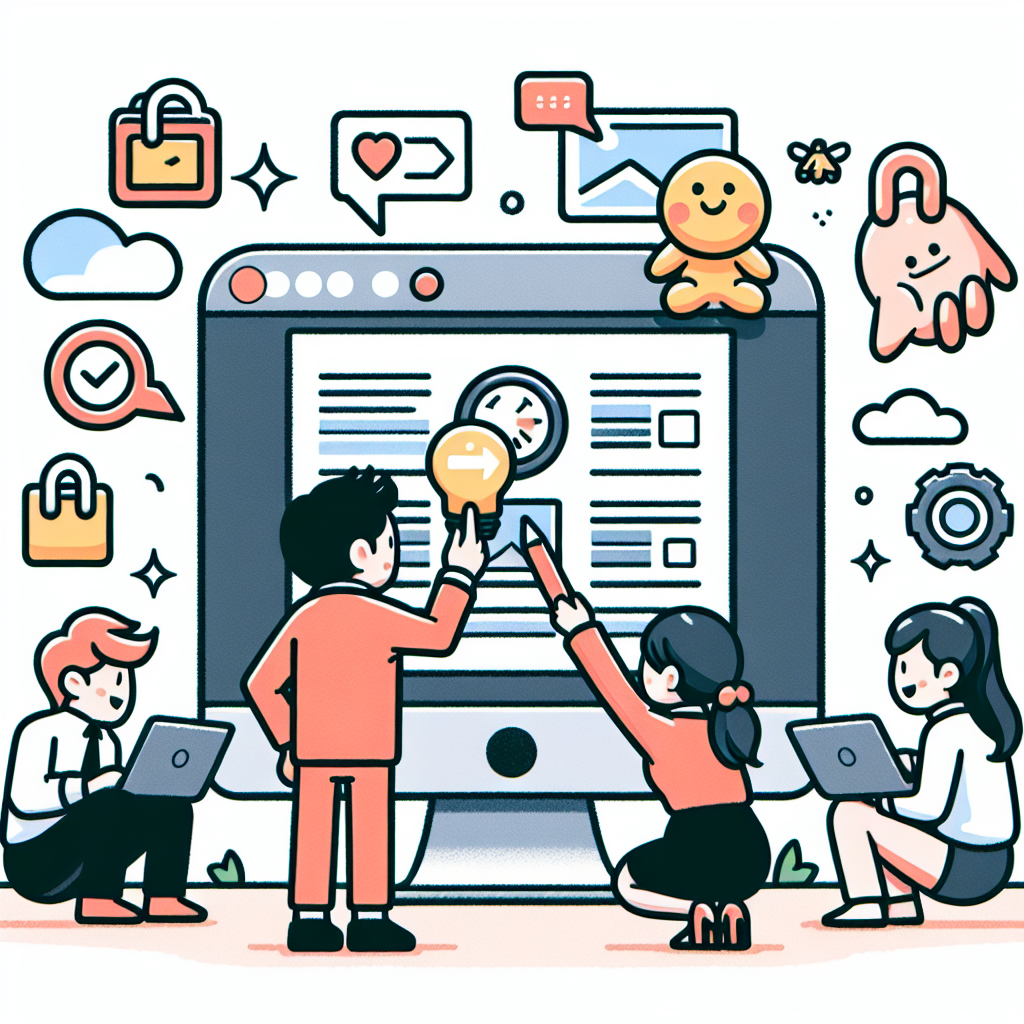
### 使用Python与BeautifulSoup
Python是一种强大且易于使用的编程语言,配合BeautifulSoup库,我们可以轻松解析网页内容并提取图片链接。
```python
import requests
from bs4 import BeautifulSoup
import os
# 指定要抓取图片的网页URL
url = 'https://example.com'
# 发送HTTP请求,获取网页内容
response = requests.get(url)
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 找到所有的<img>标签
images = soup.find_all('img')
# 创建存放图片的目录
os.makedirs('images', exist_ok=True)
# 循环遍历所有图片链接并下载
for img in images:
img_url = img.get('src')
# 处理相对路径
if not img_url.startswith('http'):
img_url = url + img_url
img_data = requests.get(img_url).content
# 提取图片文件名
img_name = os.path.join('images', img_url.split('/')[-1])
# 保存图片文件
with open(img_name, 'wb') as file:
file.write(img_data)
print(f'下载完成: {img_name}')
```
### 使用JavaScript与Node.js
如果你更喜欢使用JavaScript,可以通过Node.js来实现图片的抓取与保存。下面的例子使用`axios`和`cheerio`两个库来获取网页内容和解析HTML。
```javascript
const axios = require('axios');
const cheerio = require('cheerio');
const fs = require('fs');
const path = require('path');
// 要抓取的网页URL
const url = 'https://example.com';
axios.get(url)
.then(response => {
const $ = cheerio.load(response.data);
// 查找所有的<img>标签
$('img').each((index, element) => {
let imgSrc = $(element).attr('src');
// 处理相对路径
if (!imgSrc.startsWith('http')) {
imgSrc = url + imgSrc;
}
const imgName = path.basename(imgSrc);
const imgPath = path.join(__dirname, 'images', imgName);
axios({
url: imgSrc,
responseType: 'stream',
}).then(response => {
response.data.pipe(fs.createWriteStream(imgPath));
console.log(`下载完成: ${imgPath}`);
}).catch(err => console.error(err));
});
})
.catch((error) => {
console.error(`请求失败: ${error.message}`);
});
```
### 总结
通过以上方法,你可以实现从网页批量下载图片。无论是使用Python还是Node.js,这些工具都为你提供了强大的功能,帮助你更高效地进行数据获取与处理。在实际应用中,你可以根据需求选择合适的技术栈。