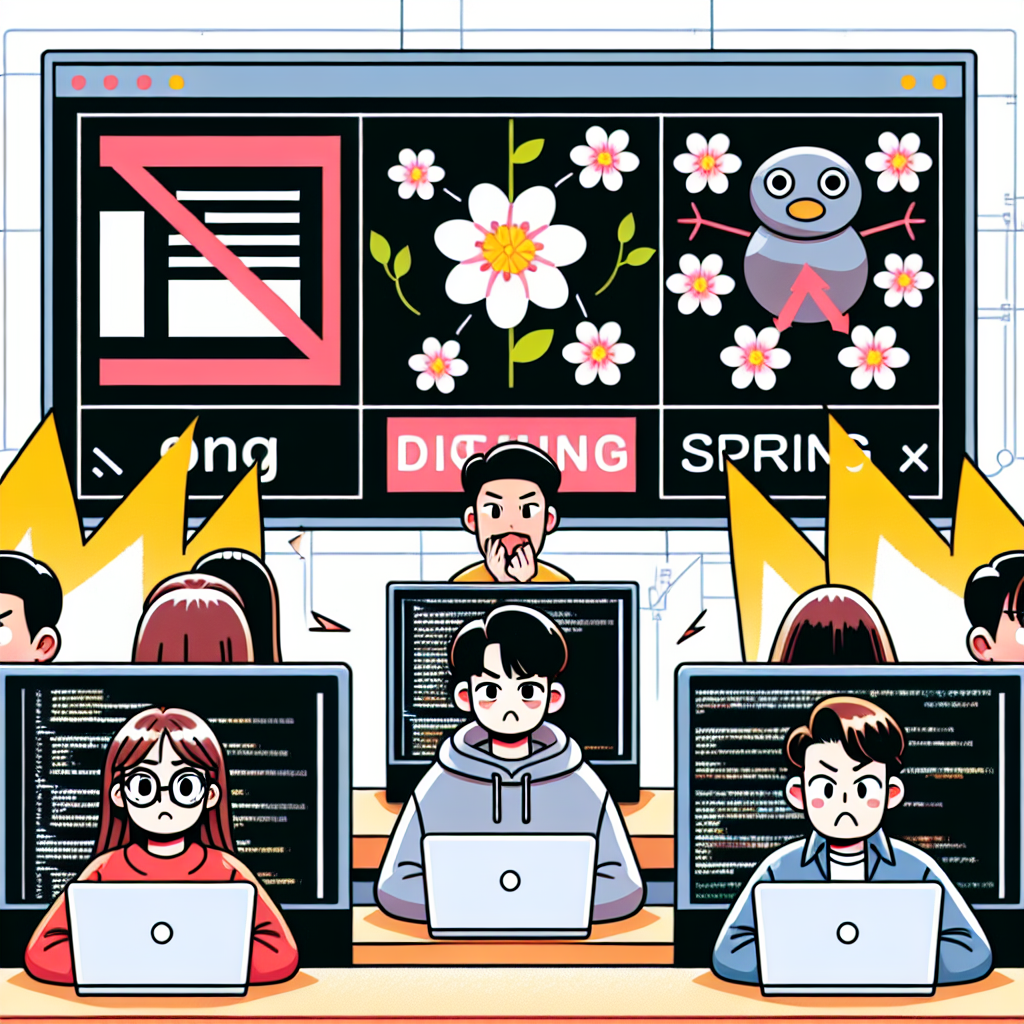
Spring Framework는 자바(Java) 기반의 어플리케이션 개발을 위한 가장 인기 있는 프레임워크 중 하나이지만, 몇 가지 단점과 한계점이 존재합니다. 여기에서는 그 중 몇 가지를 예시와 함께 설명하겠습니다.
1. 복잡성과 학습 곡선
Spring Framework는 매우 강력하고 다양한 기능을 제공하지만, 그만큼 복잡한 구조를 가지고 있습니다. 특히, 초보 개발자에게는 다소 높은 학습 곡선을 제공할 수 있습니다. 이것은 의존성 주입(Dependency Injection), AOP(Aspect-Oriented Programming)와 같은 개념들이 처음에는 이해하기 어려울 수 있기 때문입니다.
```java
// 예제: 간단한 의존성 주입
public class SimpleBean {
private Dependency dependency;
// Constructor-based Dependency Injection
public SimpleBean(Dependency dependency) {
this.dependency = dependency;
}
// Setter-based Dependency Injection
public void setDependency(Dependency dependency) {
this.dependency = dependency;
}
}
```
2. 설정의 복잡성
Spring은 유연한 설정이 가능하다는 장점이 있지만, XML이나 Java-based configuration을 모두 이해해야 하고, 프로젝트 규모가 커질수록 설정 파일의 복잡성이 증가할 수 있습니다.
```xml
<!-- XML Configuration Example -->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="simpleBean" class="com.example.SimpleBean">
<property name="dependency" ref="dependencyBean"/>
</bean>
<bean id="dependencyBean" class="com.example.Dependency"/>
</beans>
```
3. 성능 문제
Spring은 다양한 기능을 제공하여 유연성을 높이지만, 종종 런타임 시 성능 부하를 초래할 수 있습니다. 특히, 많은 빈(bean)을 사용하는 프로젝트에서는 초기 로딩 시간이 길어질 수 있으며 메모리 사용량이 증가할 수 있습니다.
```java
// 예제: 다수의 빈을 사용한 경우
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Autowired
private EmailService emailService;
// ... Additional dependencies
}
```
4. 특정 기능의 사용 어려움
Spring Framework는 모든 기능을 어렵지 않게 사용할 수 있도록 설계된 것은 아닙니다. 예를 들어, AOP를 사용하여 복잡한 cross-cutting concerns을 구현하는 것은 쉽지 않으며, 올바르게 사용하지 않으면 코드의 예측 가능성을 떨어뜨릴 수 있습니다.
```java
// 예제: AOP 사용
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("AOP Before Method: " + joinPoint.getSignature().getName());
}
}
```
Spring Framework는 풍부한 기능과 유연한 아키텍처를 제공하지만, 그에 따른 복잡성과 성능 문제, 학습의 어려움이 있을 수 있습니다. 이러한 점들을 충분히 이해하고 프로젝트에 적용하는 것이 중요합니다.